How to Configure Subscriptions on the Web with Stripe and Grant Users Access on iOS and Android
How to Configure Subscriptions on the Web with Stripe and Grant Users Access on iOS and Android

Maria

Maria
Aug 10, 2022
Aug 10, 2022
Implementing renewable subscriptions is a daunting task, especially if you haven’t dealt with a third-party payment processors’ APIs like StoreKit, Google Billing Library, Stripe before. And it becomes much harder when you need to support several platforms simultaneously. You’ll find that managing cross-platform subscriptions is challenging as you need to solve technical integration complexity, consider different platforms’ policies and requirements, find the right approach to manage subscription statuses (cancellations, upgrades, downgrades, etc.), and analytics.
At the same time, cross-platform subscription management provides your users with the best user experience on any device anywhere, and allows you to reduce platform fees (15-30% for app stores and 2-3% for Stripe — just feel the difference).
In our previous article, we covered granting access on the web after a purchase was made on iOS and Android platforms. Now let’s dig deeper into the web part and explore how to configure Stripe payments and grant your users premium access on iOS and Android apps.
Implementing renewable subscriptions is a daunting task, especially if you haven’t dealt with a third-party payment processors’ APIs like StoreKit, Google Billing Library, Stripe before. And it becomes much harder when you need to support several platforms simultaneously. You’ll find that managing cross-platform subscriptions is challenging as you need to solve technical integration complexity, consider different platforms’ policies and requirements, find the right approach to manage subscription statuses (cancellations, upgrades, downgrades, etc.), and analytics.
At the same time, cross-platform subscription management provides your users with the best user experience on any device anywhere, and allows you to reduce platform fees (15-30% for app stores and 2-3% for Stripe — just feel the difference).
In our previous article, we covered granting access on the web after a purchase was made on iOS and Android platforms. Now let’s dig deeper into the web part and explore how to configure Stripe payments and grant your users premium access on iOS and Android apps.
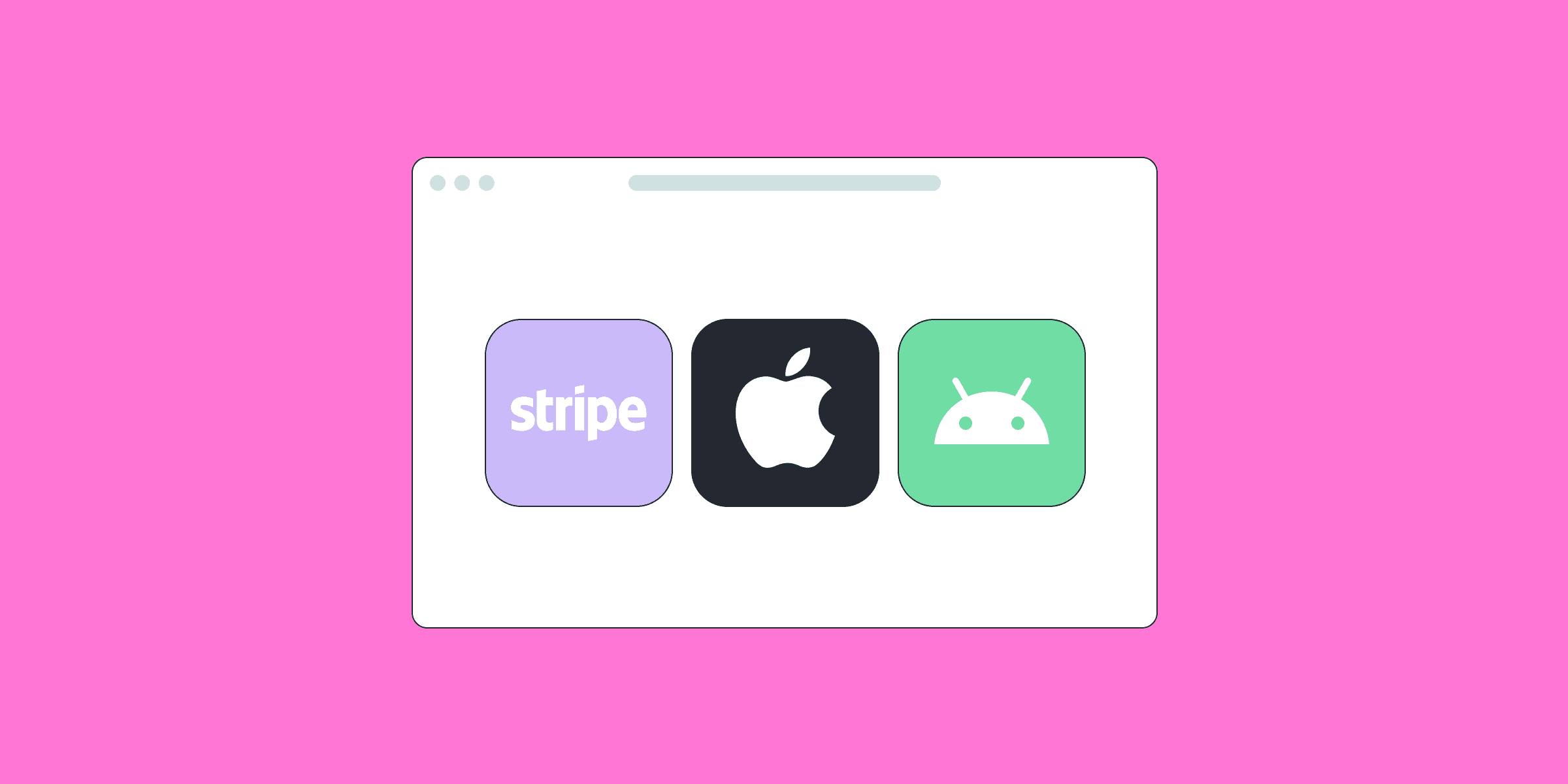
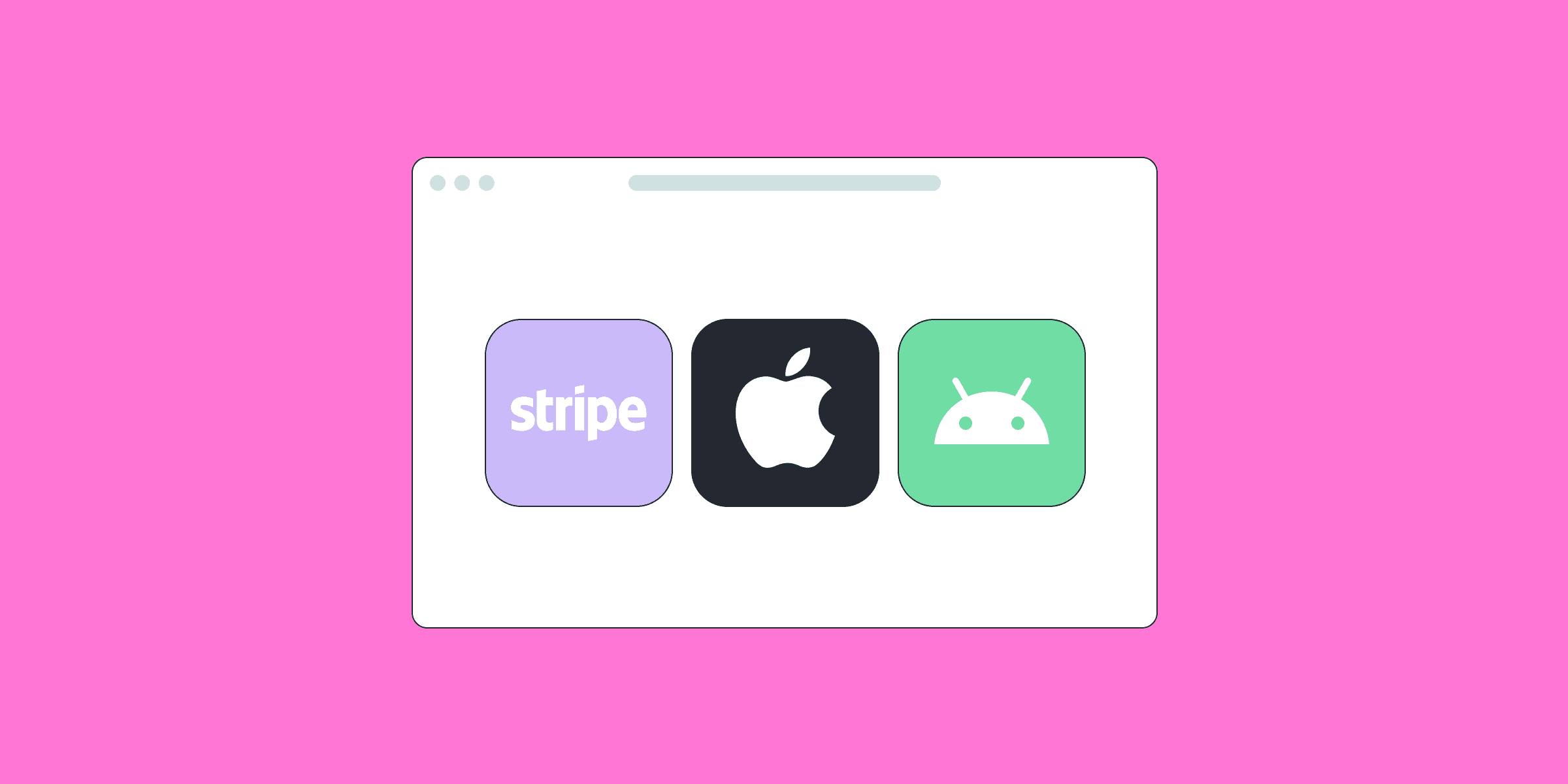
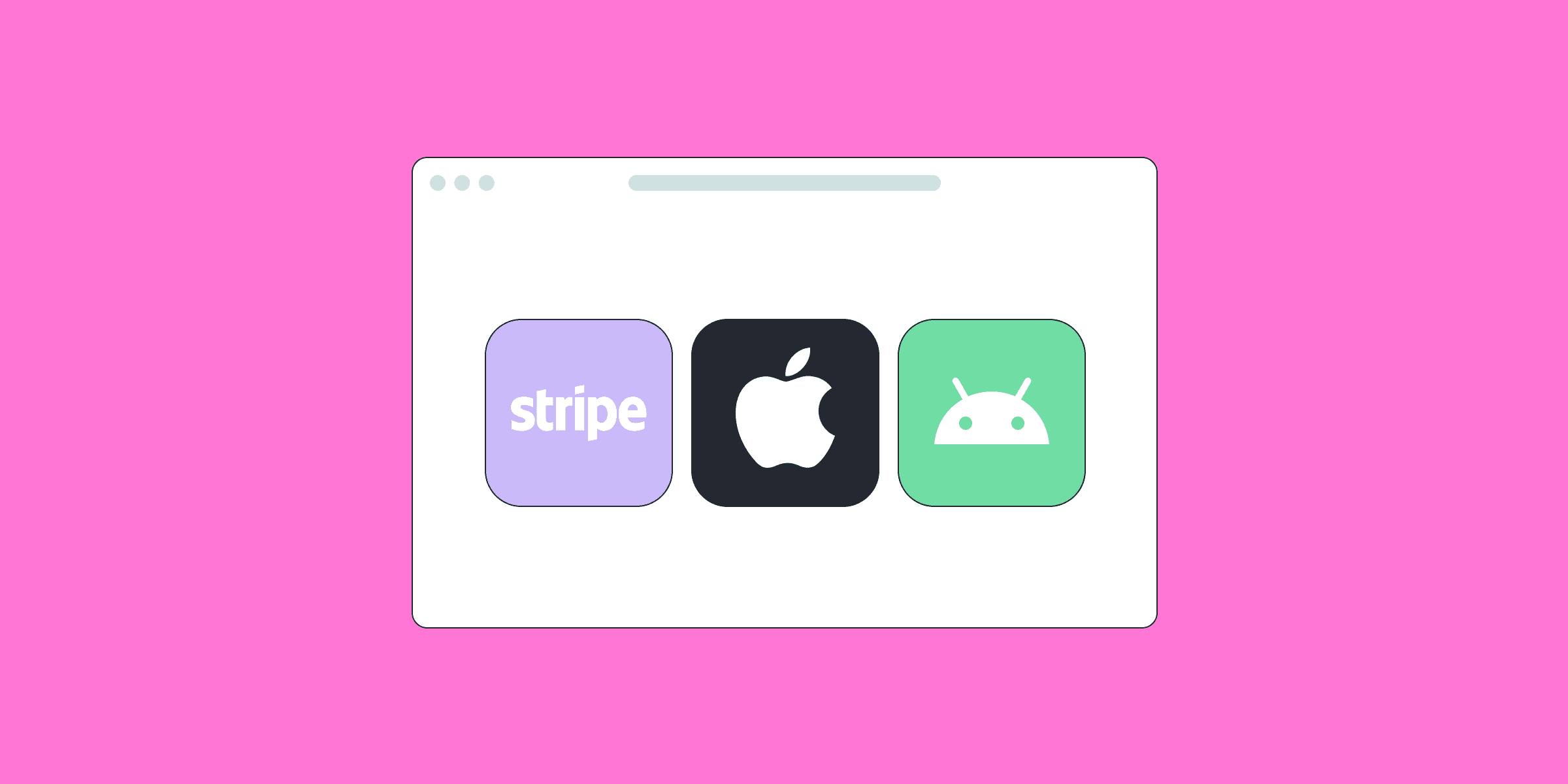
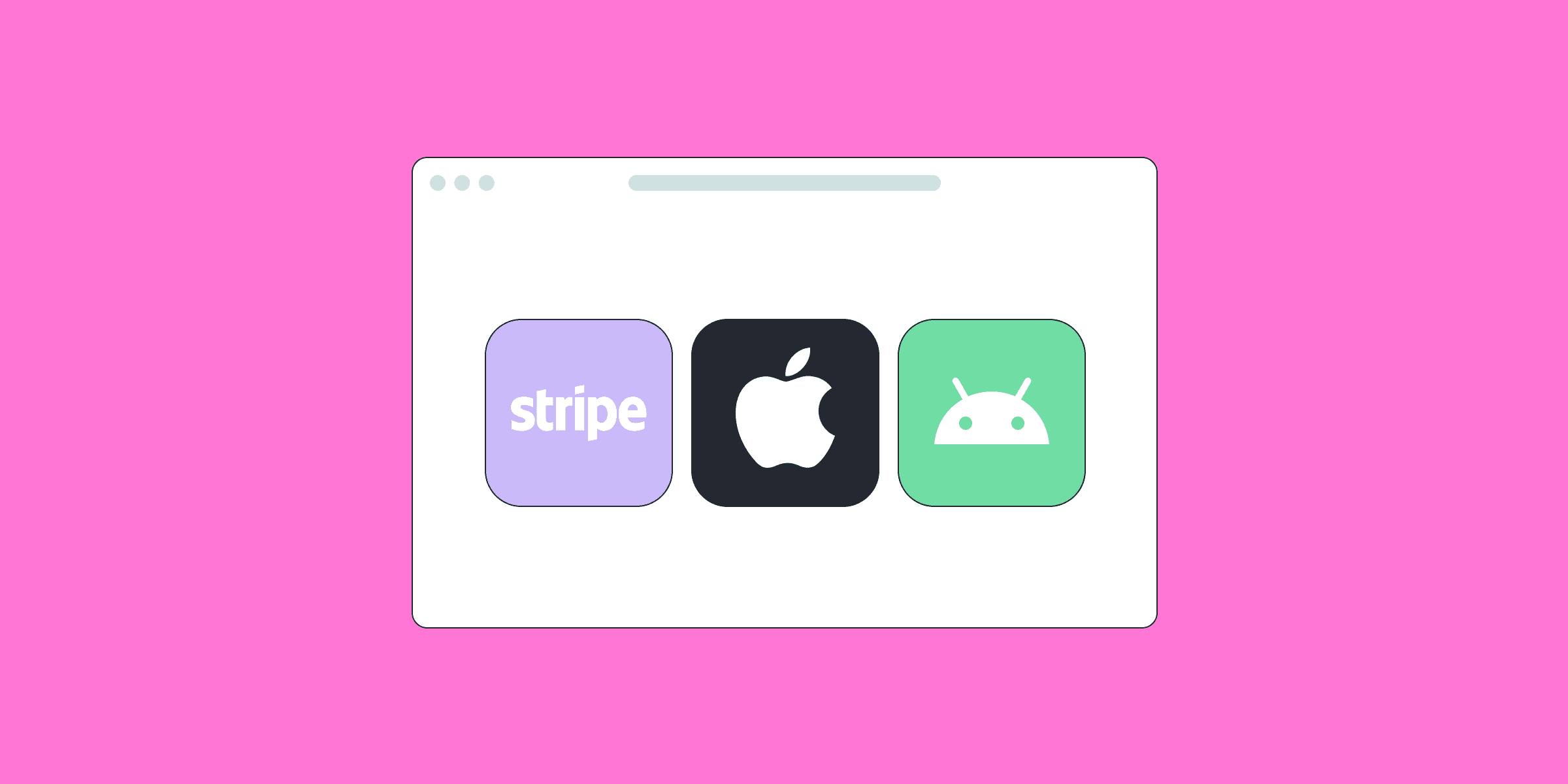