Summary
A Deep Dive into StoreKit 2 Capabilities
A Deep Dive into StoreKit 2 Capabilities

Suren

Suren
Jun 30, 2021
Jun 30, 2021
During WWDC21, Apple announced many exciting product features, and StoreKit 2 is one of the most significant updates. In-app subscriptions have become the most popular monetization method that generates billions of dollars in revenue for Apple and thousands of mobile app developers. Developers have earned $230 billion through the App Store since its launch, with the biggest chunk of that revenue coming in the last few years.
That makes in-app purchases a particular focus of Apple and Google. Just last year WWDC20 presented StoreKitTransactionManager with a .storekit file that simplifies the process of in-app purchases testing. We have been waiting for this update since iOS3. And the next updates were not long in coming.
This article will cover what’s new in StoreKit 2 and discuss the difference between the updated framework and its previous version – StoreKit.
Before we jump into StoreKit 2 overview, let’s briefly cover what was wrong with the previous StoreKit.
During WWDC21, Apple announced many exciting product features, and StoreKit 2 is one of the most significant updates. In-app subscriptions have become the most popular monetization method that generates billions of dollars in revenue for Apple and thousands of mobile app developers. Developers have earned $230 billion through the App Store since its launch, with the biggest chunk of that revenue coming in the last few years.
That makes in-app purchases a particular focus of Apple and Google. Just last year WWDC20 presented StoreKitTransactionManager with a .storekit file that simplifies the process of in-app purchases testing. We have been waiting for this update since iOS3. And the next updates were not long in coming.
This article will cover what’s new in StoreKit 2 and discuss the difference between the updated framework and its previous version – StoreKit.
Before we jump into StoreKit 2 overview, let’s briefly cover what was wrong with the previous StoreKit.
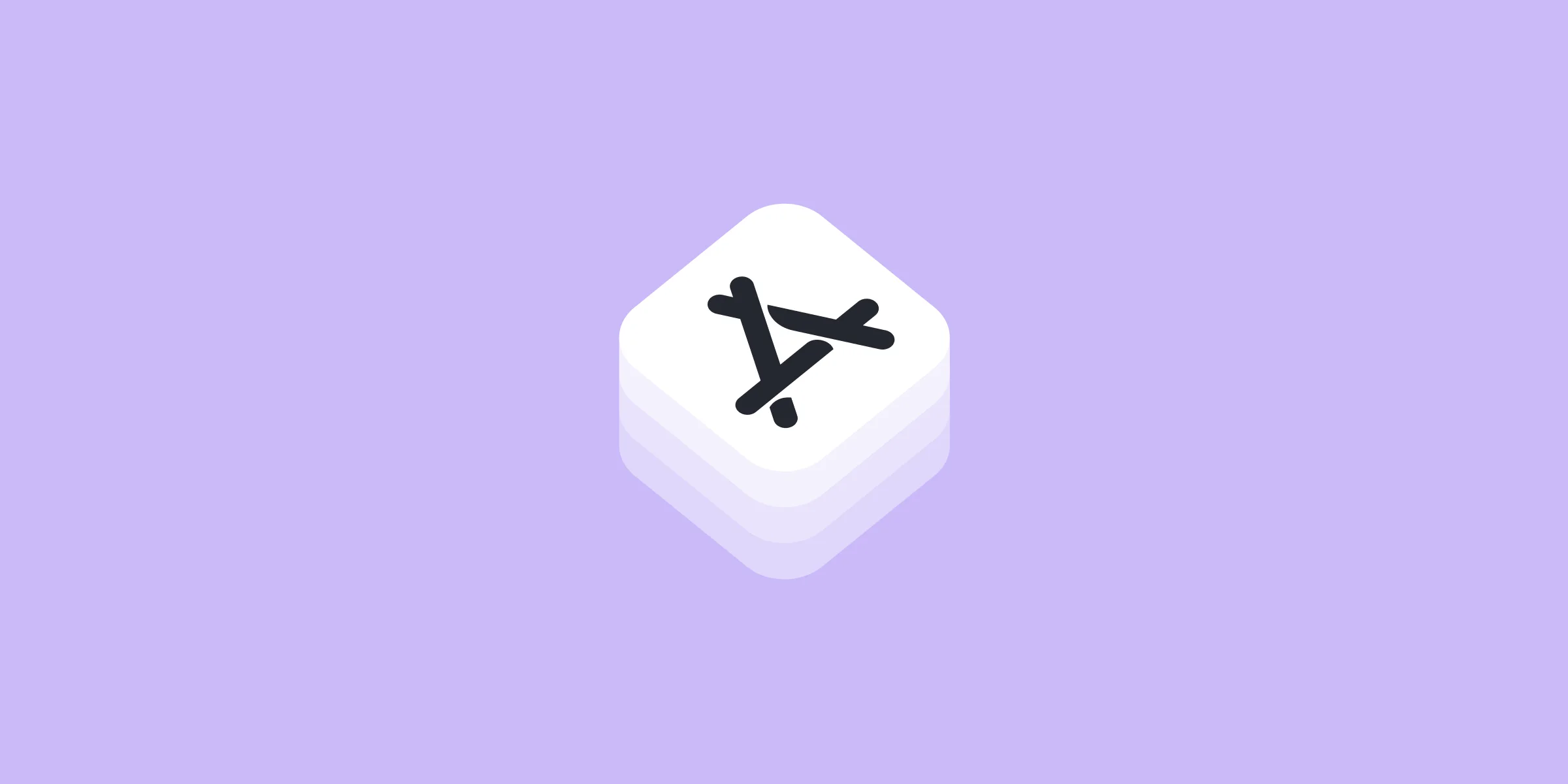
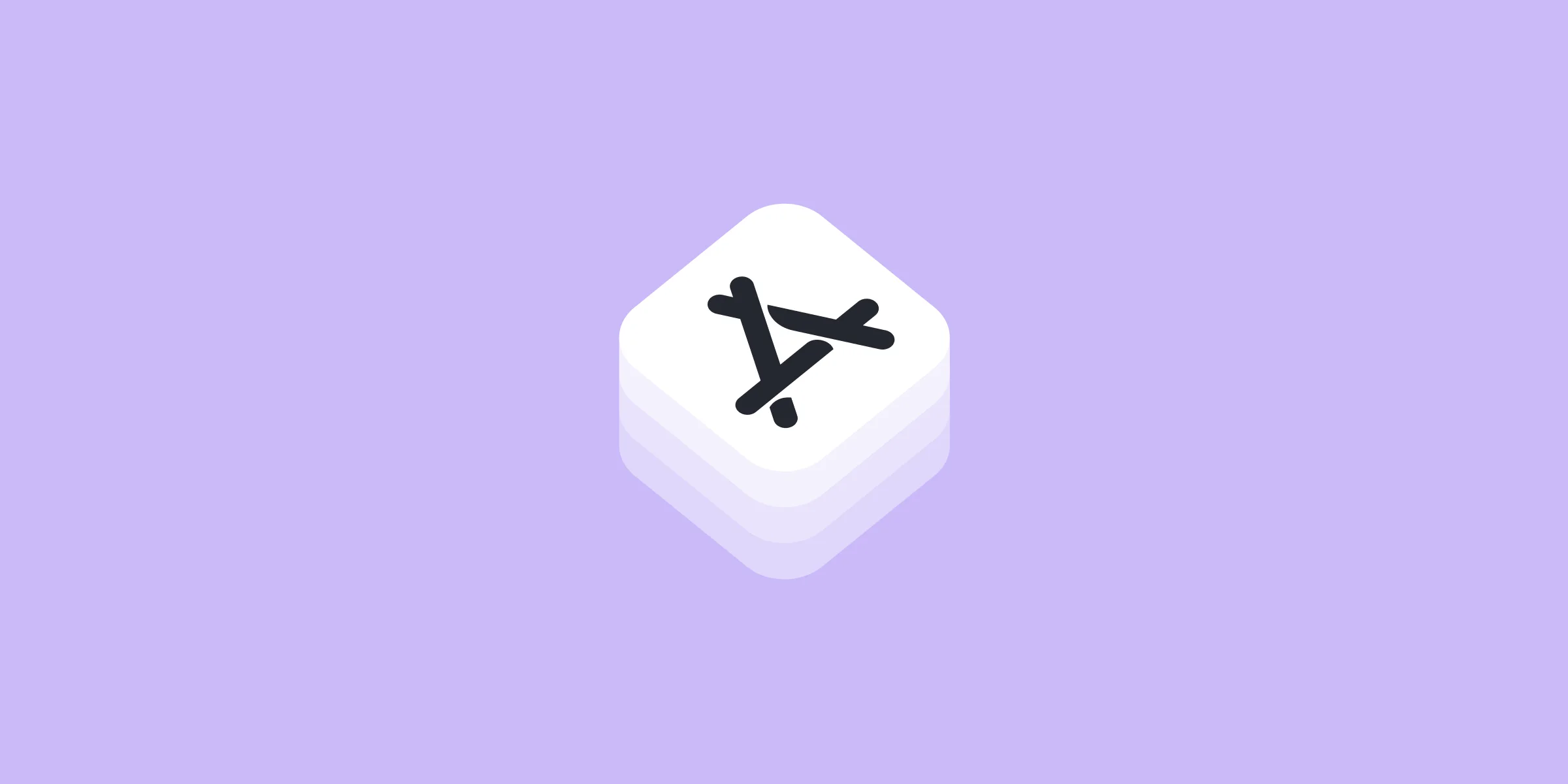
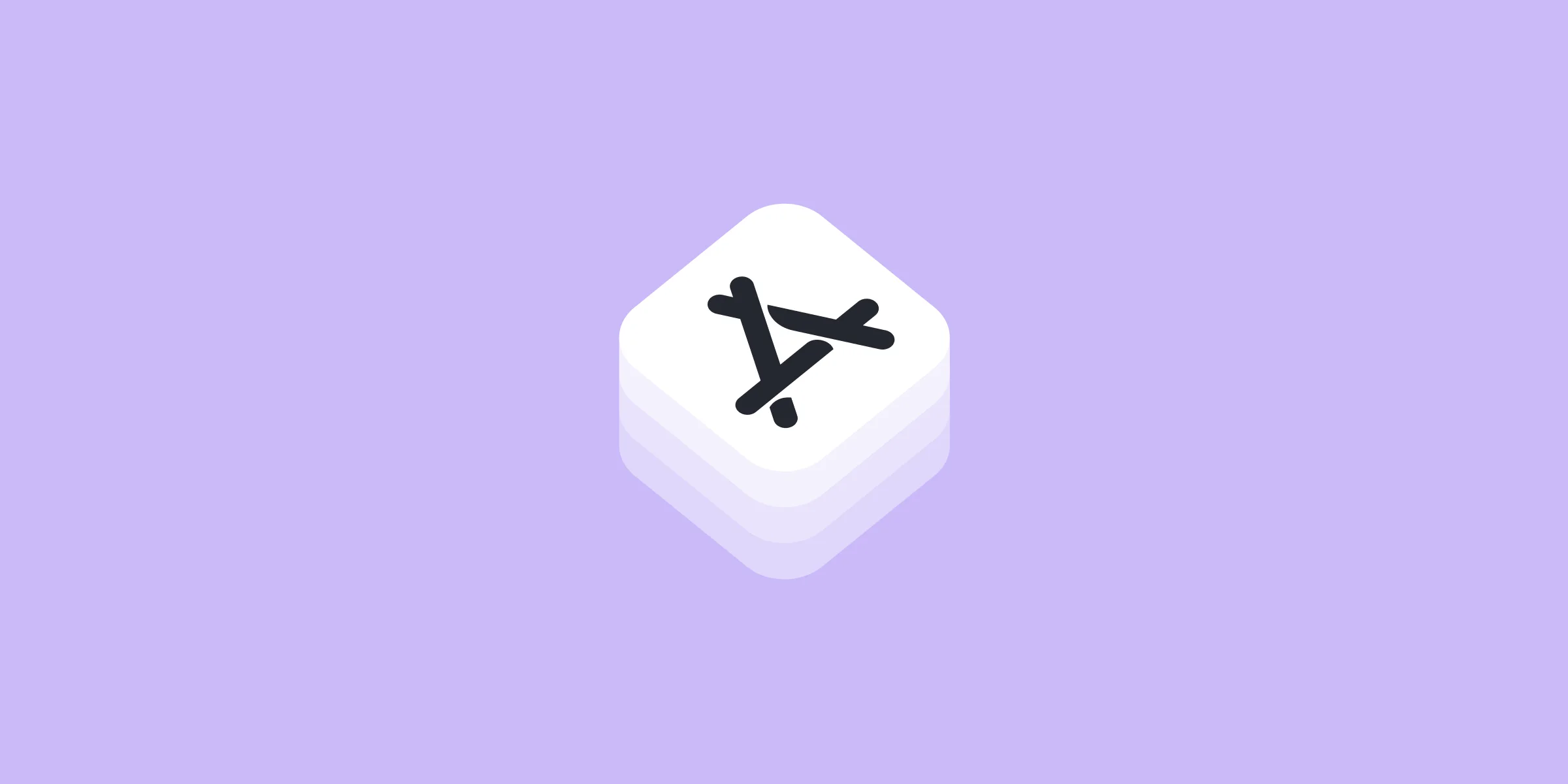
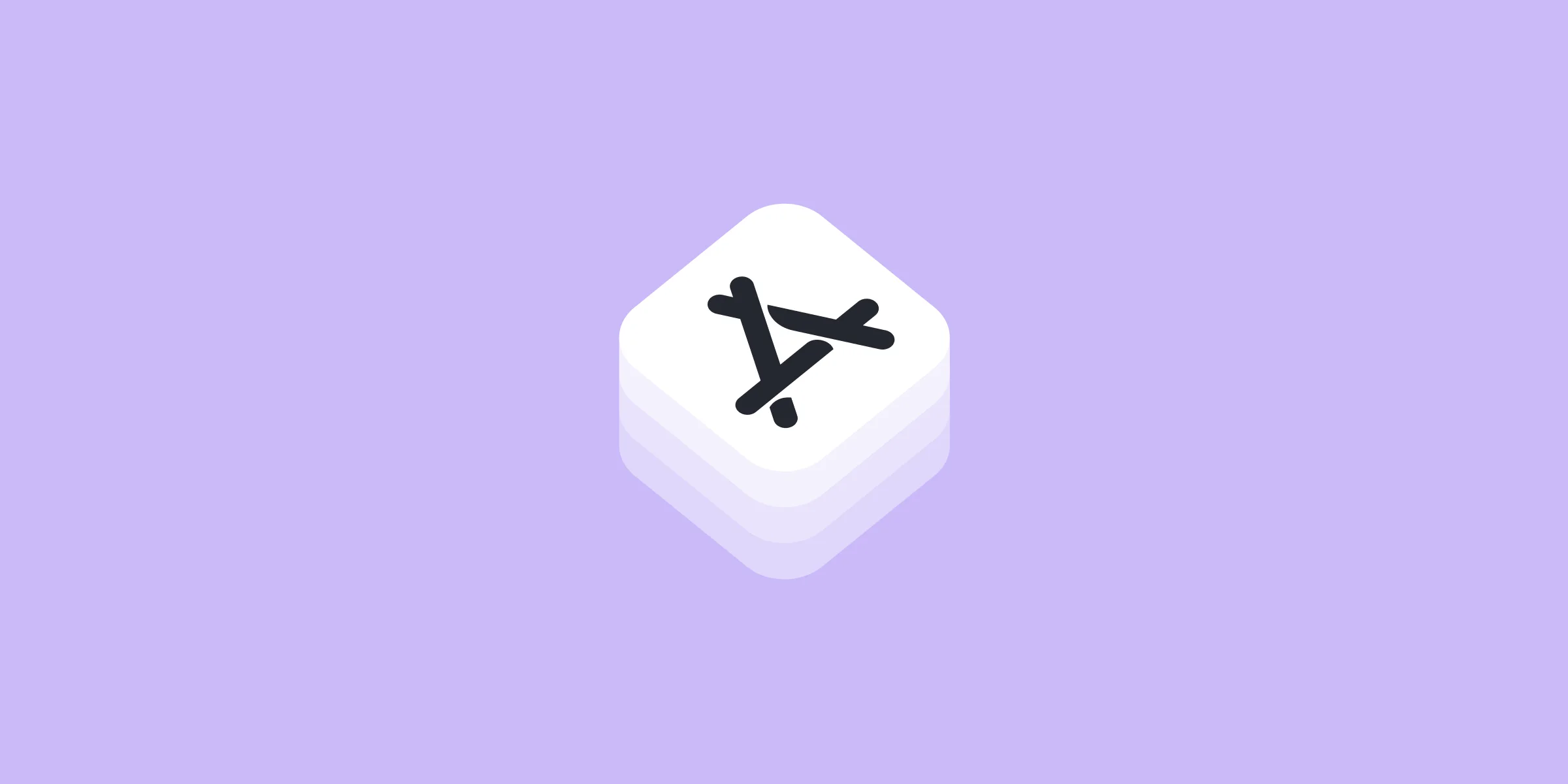
Start Now for Free
Or book a demo with our team to learn more about Qonversion
Start Now for Free
Or book a demo with our team to learn more about Qonversion
Start Now for Free
Or book a demo with our team to learn more about Qonversion
Read more
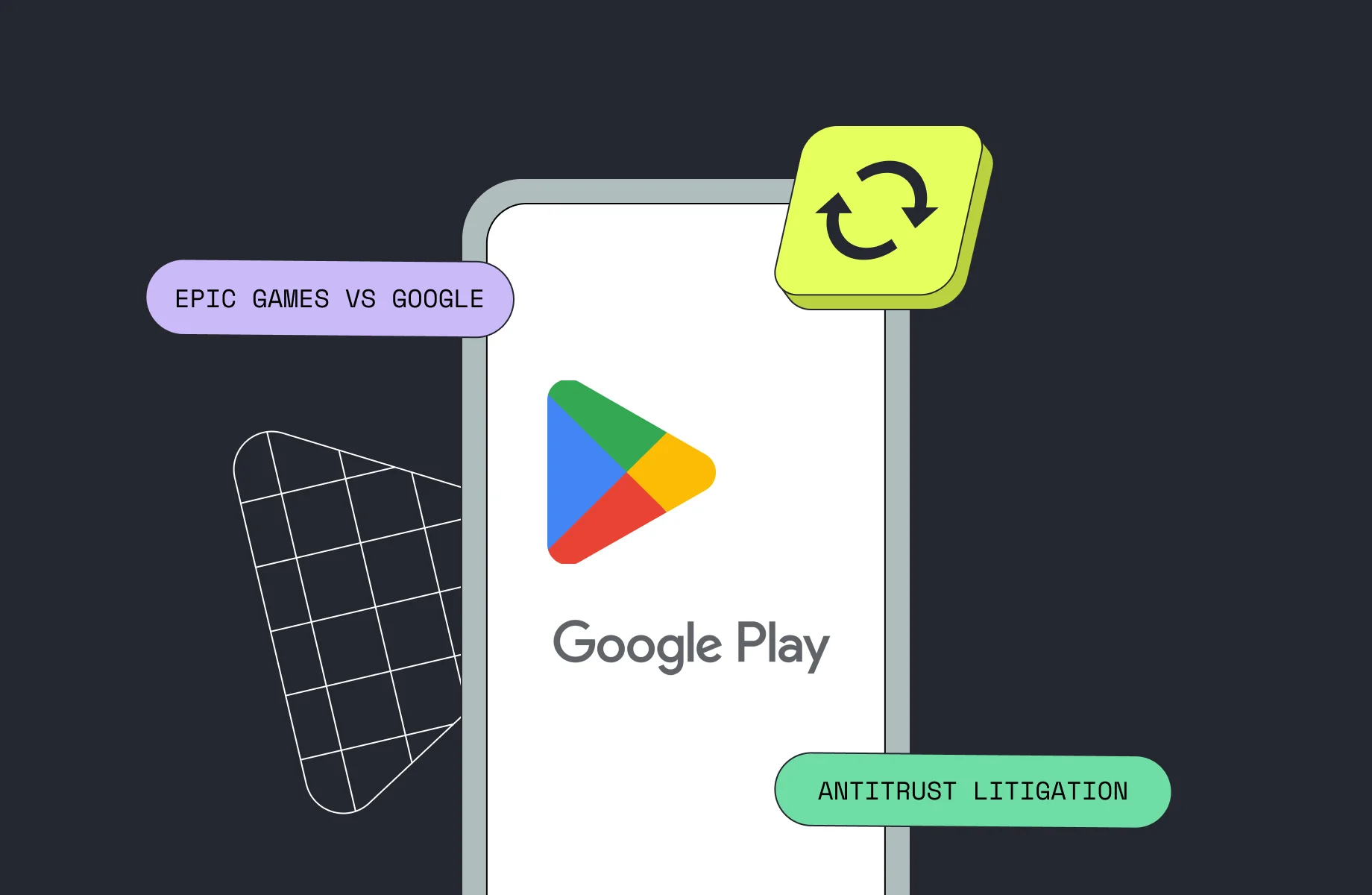
How will Google Play Store Lawsuit on Monopoly Impact Play Store Commissions and Other Marketplaces?
Oct 10, 2024
Oct 10, 2024
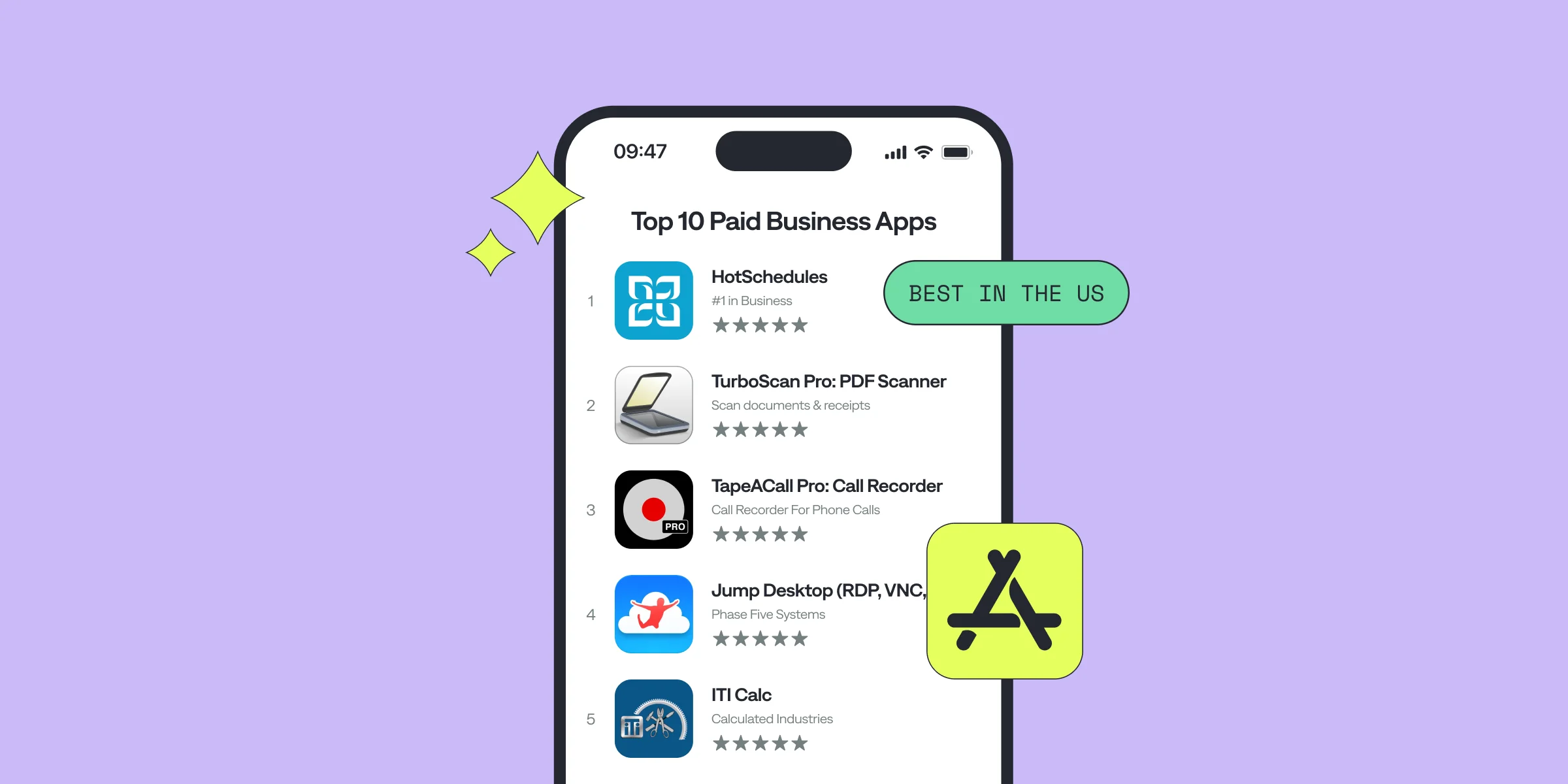
Top 10 Paid and Subscription Apps in Business Category
Oct 9, 2024
Oct 9, 2024
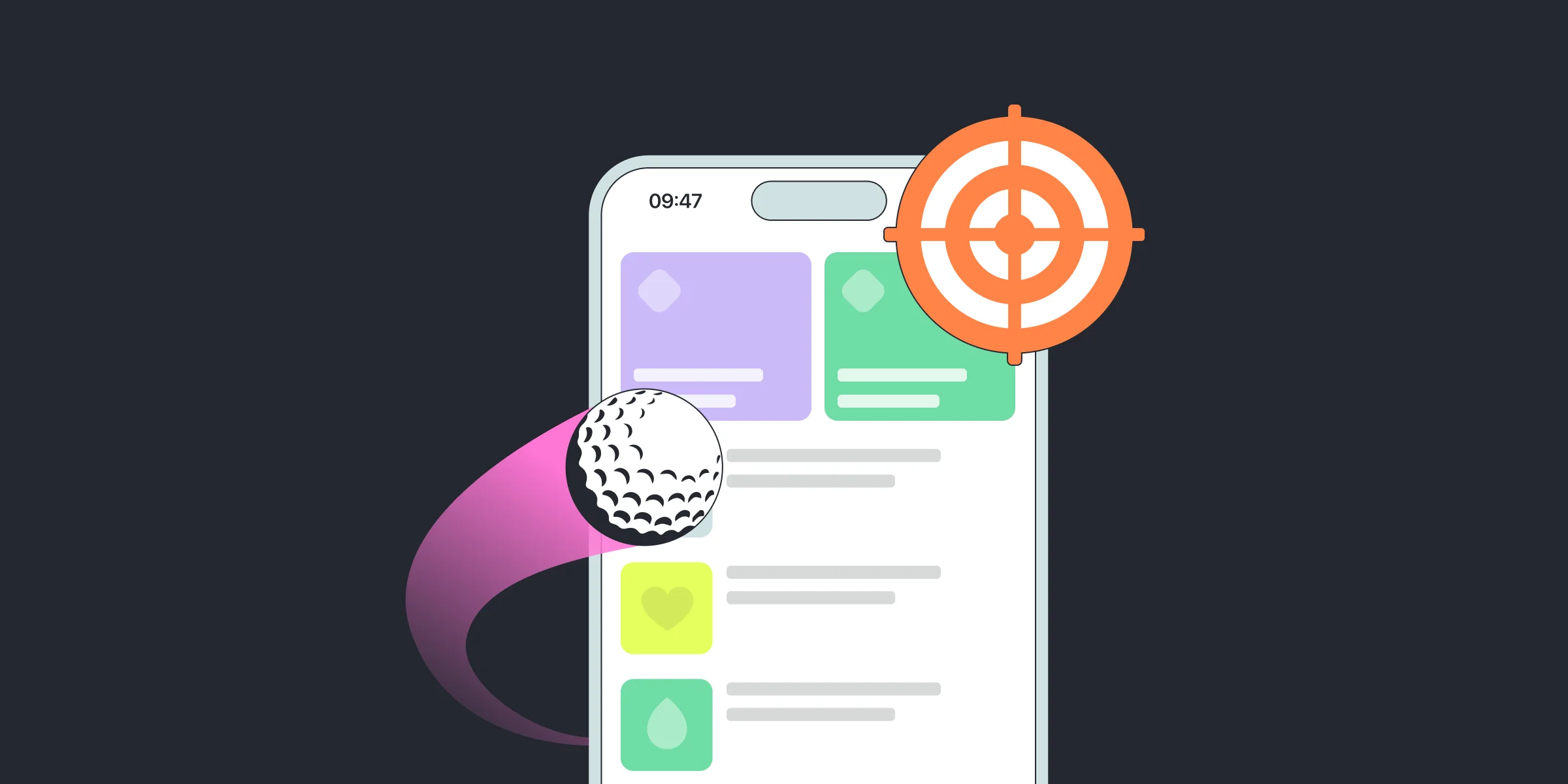
Top 10 Paid Apps in Sports According to the App Store
Sep 27, 2024
Sep 27, 2024

All on Apple Fiscal Calendar: 2025 Payout Dates
Sep 13, 2024
Sep 13, 2024